Player Movement:
Coding the player movement for Pong is an easy task. When the 'w' key is pressed, the player needs to move up (subtract from the y position). When the 's' key is pressed, the player will move down (add to the y position).
pygame.key.get_pressed() - this function returns a list of all the keyboard keys and their key states (true if the key is pressed, false if not)
pygame.K_w - the pygame code for the 'w' key. Here, the K is short for key
pygame.K_s - the pygame code for the 's' key.
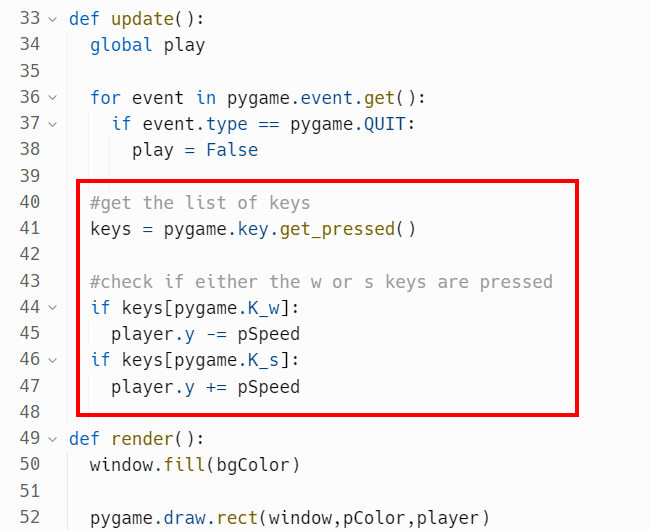
When you press w or s, you may notice the player paddle moves incredibly quickly. However, this is not because the player's speed is big. The player is moving too fast because the game is currently trying to run as fast as possible. We only need it to run at about 60 fps (frames per second). To do this, we need to create a Pygame clock and use its tick function.
pygame.time.Clock() - this function creates a Pygame clock for us to use
[clock variable].tick(target_fps) - this function controls the fps of the game. It takes your target fps as an argument.
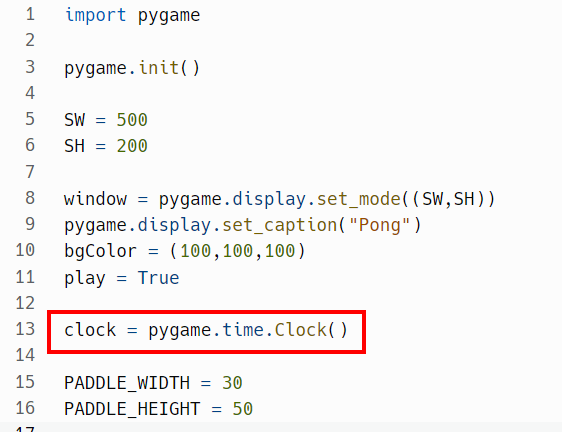
From inside the update function, we limit the game speed to 60 fps.
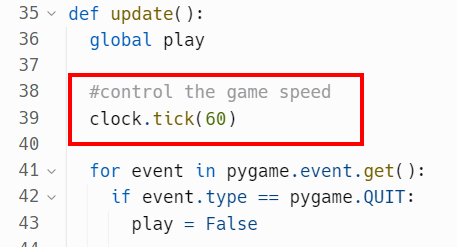
At this point, the player should be moving at a much more reasonable speed.
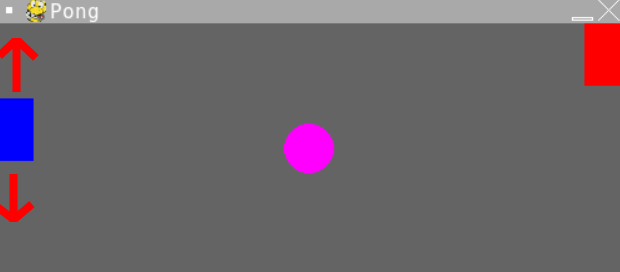
Staying in Bounds:
Let's make sure that the player can't go off-screen. To keep the player paddle from going too high, we need to make sure it's y can't be less than zero
Note: the player position is always the top left corner of the paddle, NOT the middle of the paddle.
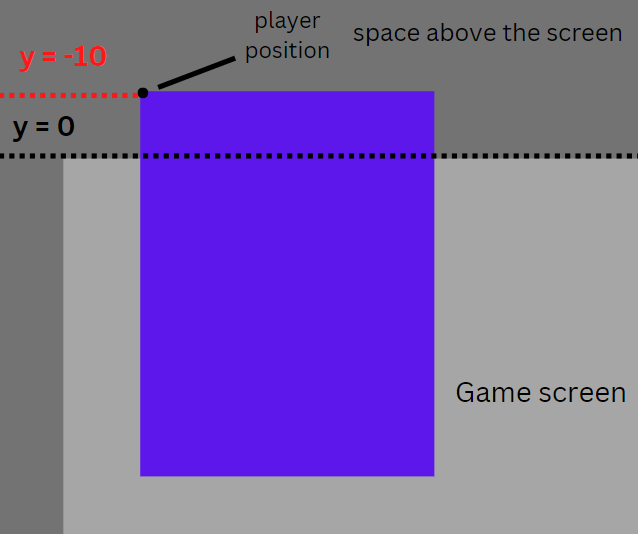
To keep the player from going too low, we make sure that player y+ paddle height stays less than the screen height.
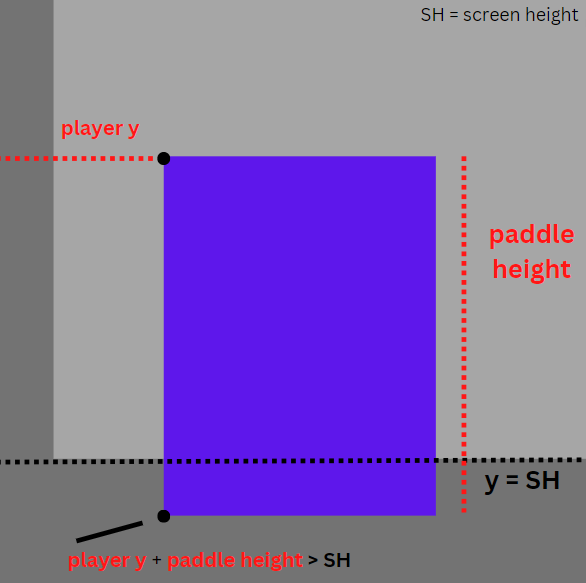
In practice, the code will look like this.
Note: player.h is the paddle height.
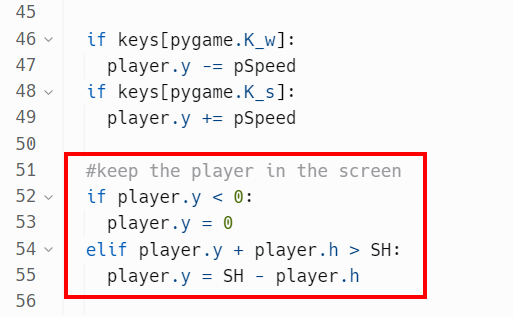
Computer Movement:
Our AI for the computer paddle will be simple. If the ball is higher than the paddle, the paddle will move up, and if the ball is lower, the paddle will lower. This is the simplest way to get the computer to track the ball.
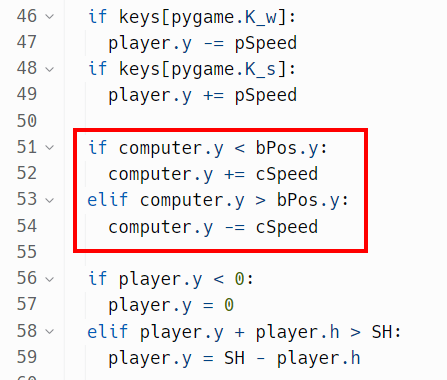
The code to keep the computer paddle inside the screen uses the exact same logic for keeping the player inside the screen.
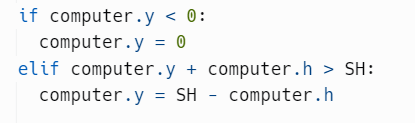
Now when you start the game, the computer paddle will move down so it is across from the ball.
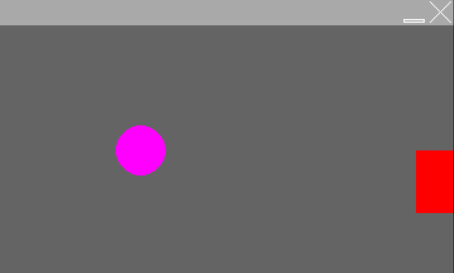